Calculating length of a Span of bytes when encoding text [Answered]
I need a method that takes text, encodes it and converts it to base 64 without allocations (if possible).
This is what I have so far:
I'm using this to encode/decode JSON. I have a unit test for roundtripping (encode/decode the same object and check it's equivalent) this that is failing.
It decodes the actual JSON correctly, but then continues onwards, filling up the string with garbage data that causes deserialization to explode.
{"Name":"John Doe","Age":32,"Alive":truep4��bY,P�&��4]�!�V��!�]D��aQ<@0XbUh2VaplSHNXUThGbhhUTwQQP==
11 Replies
My assumption is that I'm messing up how big the
Span<byte>
should be and that's causing it to include garbage data, but I don't see what I'm doing wrong.
I am doing other stuff besides just encoding the JSON that I can explain if all of that looks OK, but wanted to check this first since I've not messed with spans before.check the Span<byte>'s [length] to make sure its null terminated
doesn't appear so
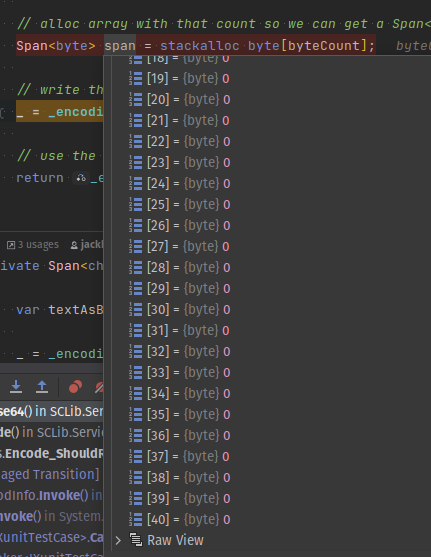
should I set its length to byteCount + 1 and ensure the last element is
null
?yeah
what's the correct syntax for that -
span[^1] = null;
complains that I can't assign null to byte
or is it fine for it to be a Span<byte?>
?0
ah, got you - makes sense
tried that and i'm still getting garbage data added in so i think i need to check the rest of my implementation
figured it out, in my method where I parsed the base64, I was writing back into the same span<char> I was reading from 😄
do /close
mhmm
✅ This post has been marked as answered!