What's a repository and the repository pattern? [Answered]
Some articles are telling me that a repository is in essence just an interface that abstracts data access such that it doesn't what the underlying database is. I know there's usually an
IWhateverRepository
interface for a repository which just defines methods for enumerating and getting objects. Is this all there is to it?
"The idea behind the Repository pattern is to decouple the data access layer from the business access layer of the application so that the operations (such as adding, updating, deleting, and selecting items from the collection) is done through straightforward methods without dealing with database concerns such as connections, commands, and so forth."46 Replies
that's a decent description, what else do you want to know
No I was just wondering, sounds too simple to be true.
consider if you didn't have an access layer, you're going to mix SQL and db connections up with your business logic, and you're going to have a bad complexity problem
especially if you have a lot of models or your logic is non-trivial
also consider unit/integration testing
Yeah that sounds... horrible
the usual recommendation around here is to use Entity Framework, which gives you a repository pattern, and unlike the typical "repository pattern example" you see when you search the internet...allows you to write LINQ queries to filter your datasets directly, and translates those to SQL for you
So the intention is to allow you to integrate EF directly with your business logic?
pretty much
How would that allow you to switch implementations?
I haven't actually ever used EF so I know barely anything, but my intention is to eventually learn it.
switching from EF to a different ORM framework basically doesn't happen in practice, although some like to hide it behind an interface to allow for unit testing
Yeah, that sounds like the one major benefit
patrickk#0001
OK SO..........
When you're using Entity Framework, especially EFCore, there is no real need for a repository because EF already is a repository. While previous versions of EF were the same, it was incredibly hard to "mock" or test the use of Entity Framework in classes because it would end up making a real database call, which is undesirable in tests. People came up with the idea of coupling EF with the repository pattern to make testing easier. This has the """"bonus""" effect of abstracting EF. This is where your problems begin. Because repositories are written by you, you're effectively massively dumbing down EF. You cannot use any of its "powerful" APIs outside of the repository for specialized purposes and often developers will then limit themselves to the holy grail of shit that are generic repositories. EF already is a repository. You're writing a repository over a repository. Once you have that repository written, you end up needing to solve the problem of the db context scope - which we might refer to as a unit of work. EF implements a unit of work. EF has a unit of work that's more than capable of providing what you require; HOWEVER, once you abstract EF away under a repository you lose that as well, so suddenly you need to write your own unit of work mechanism, slowly rewriting aspects of EF that you could just be using directly to make both your and your app's life easier and better. Finally the immense layering and baggage that comes with the repository pattern just ends up impeding any time you could be spending doing something valuable, like getting your app to work. This isn't a rant against the repository pattern, this is a rant against using the repository pattern over EF, especially EF Core. Please don't hate me MODiX devs.
Quoted by
<@!98435905005572096> from #modix-development (click here)
React with ❌ to remove this embed.
or search for "repository over ef" for similar discussions
if you're interested in testing, you're better off using real EF and possibly swapping out the provider for SQLite or the In-Memory thing
I wanna eventually integrate an SQLite DB into this project using EF, but for now I'm just gonna use an in-memory thing. Is that a bad idea?
@Trinitek
I don't think the in-memory provider is intended for production use
why don't you want to persist your data?
Because right now I want to get used to creating web APIs and I don't want to try integrate a full-on DB yet.
I'm not making anything intended for serious "production"
just use SQLite, you'll have less surprises
Got any good resources on getting started with it? I found think video by Tim Corey and I hope it's at least decent.
https://www.youtube.com/watch?v=ayp3tHEkRc0
IAmTimCorey
YouTube
Using SQLite in C# - Building Simple, Powerful, Portable Databases ...
Have you ever wanted to store data for an application but didn't want to deal with the hassle of a full database server? Or maybe you wanted to have one database per installation. Those and many other scenarios are a perfect fit for SQLite. Today I am going to show you how to get started using SQLite in C#. We will build a small database, attach...
description mentions Dapper instead of EF, avoid
oof
https://docs.microsoft.com/en-us/aspnet/core/data/ef-rp/intro?view=aspnetcore-6.0&tabs=visual-studio you can trust this one to be up-to-date
Razor Pages with Entity Framework Core in ASP.NET Core - Tutorial 1...
Shows how to create a Razor Pages app using Entity Framework Core
https://docs.microsoft.com/en-us/ef/core/ and the EF docs themselves
Overview of Entity Framework Core - EF Core
General introductory overview of Entity Framework Core
nice
https://docs.microsoft.com/en-us/ef/core/providers/sqlite/limitations SQLite has some select limitations also, but I expect the tutorial to play nice
SQLite Database Provider - Limitations - EF Core
Limitations of the Entity Framework Core SQLite database provider as compared to other providers
Does this tutorial actually go through setting up SQLite?
looks like they're actually using SQL Server LocalDB instead
On the VS tab at least, the VSCode one uses SQLite
ah
Welp, guess I'm spending today learning SQLite 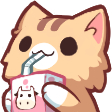
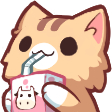
the provider is from the nuget package Microsoft.EntityFrameworkCore.Sqlite, which appears to already be included in the scaffolded example project
so for sqlite, that's all you need to do to set that up, plus setting the connection string, which is just the filename
nice
Also SQLite doesn't install a ton of extra stuff, right? Or like anything that's really hard to get rid of
SQLite itself is a single native DLL
you'll get that as part of the nuget package
Oh, so I don't even need to install SQLite?
wait no i still need it to create the actual db lmao
nope nothing to install
Wait so how do I create the DB file? Or do I just create a file and set that as the connection string and everything else is handled by SQLite/EF?
EF will create the file if it doesn't exist
or SQLite will, rather
ah, wow
That sounds so ridiculously simple
in the "seed the database" section on the "Get started" page there is a call to
context.Database.EnsureCreated()
or if you do migrations which is covered later, you'd run ApplyMigrations()
or whatever it's called exactly
yeah slightly misremembered, it will actually error if it doesn't exist
I'm thinking that's configurable as part of the connection string arguments however...Okay so what I'll need is EF Core and the SQLite EF provider Nuget packages?
yeah
actually I think if you just include the SQLite package it will bring in EF with it
not 100% sure
Nuget packages typically work like that afaik
Also we're hilariously far off-topic at this point, but thanks so much for the help
all good
@thinker227 SQLite is entirely in-process
There's no extra setup or deployment to do
ah, nice
But you can still deploy it if you want?
it will be included with the rest of your DLL dependencies, yes
I'll move to #web btw for other questions
er #database
✅ This post has been marked as answered!