how to create a jwt for an existing user in identity
I am looking to create a new JWT and validate it... for using tiwht signalr. so i want to know how to fill these fields...
here is the token generation method so what do I use in Jwt:Issuer Jwt:Audience?
33 Replies
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
You will first need to configure
Jwt&Identity
in ServiceCollection
. After that you will need to generate a Jwt for a specified User.Here is how configuration of
Jwt&Identity
could look like https://github.com/WaadSulaiman/SocialMedia.Api/blob/main/SocialMedia.Infrastructure/DependencyInjection/IdentityRegistrant.csGitHub
SocialMedia.Api/IdentityRegistrant.cs at main · WaadSulaiman/Social...
A social media api using the onion architecture. You can interact with other users by posting, commenting, following and messaging. - SocialMedia.Api/IdentityRegistrant.cs at main · WaadSulaiman/So...
Here is how you can generate a
Jwt
https://github.com/WaadSulaiman/SocialMedia.Api/blob/main/SocialMedia.Infrastructure/Helpers/TokenHandler.csGitHub
SocialMedia.Api/TokenHandler.cs at main · WaadSulaiman/SocialMedia....
A social media api using the onion architecture. You can interact with other users by posting, commenting, following and messaging. - SocialMedia.Api/TokenHandler.cs at main · WaadSulaiman/SocialMe...
do I need to set the audience?
i assume not
this is close to what i have
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
i will just use the id and normalized email
can be the issuer the assembly id?
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
yeah i thought btw
is it possible to combine JWT and cookie auth?
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
i cant get my identity to show up
when i have cookie session
it is like JWT overrides it
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
yeah i know but by enabling JWT
in authentication
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
it doesnt allow to keep the cookies
in my session
my identity user is gone
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
even after i log in
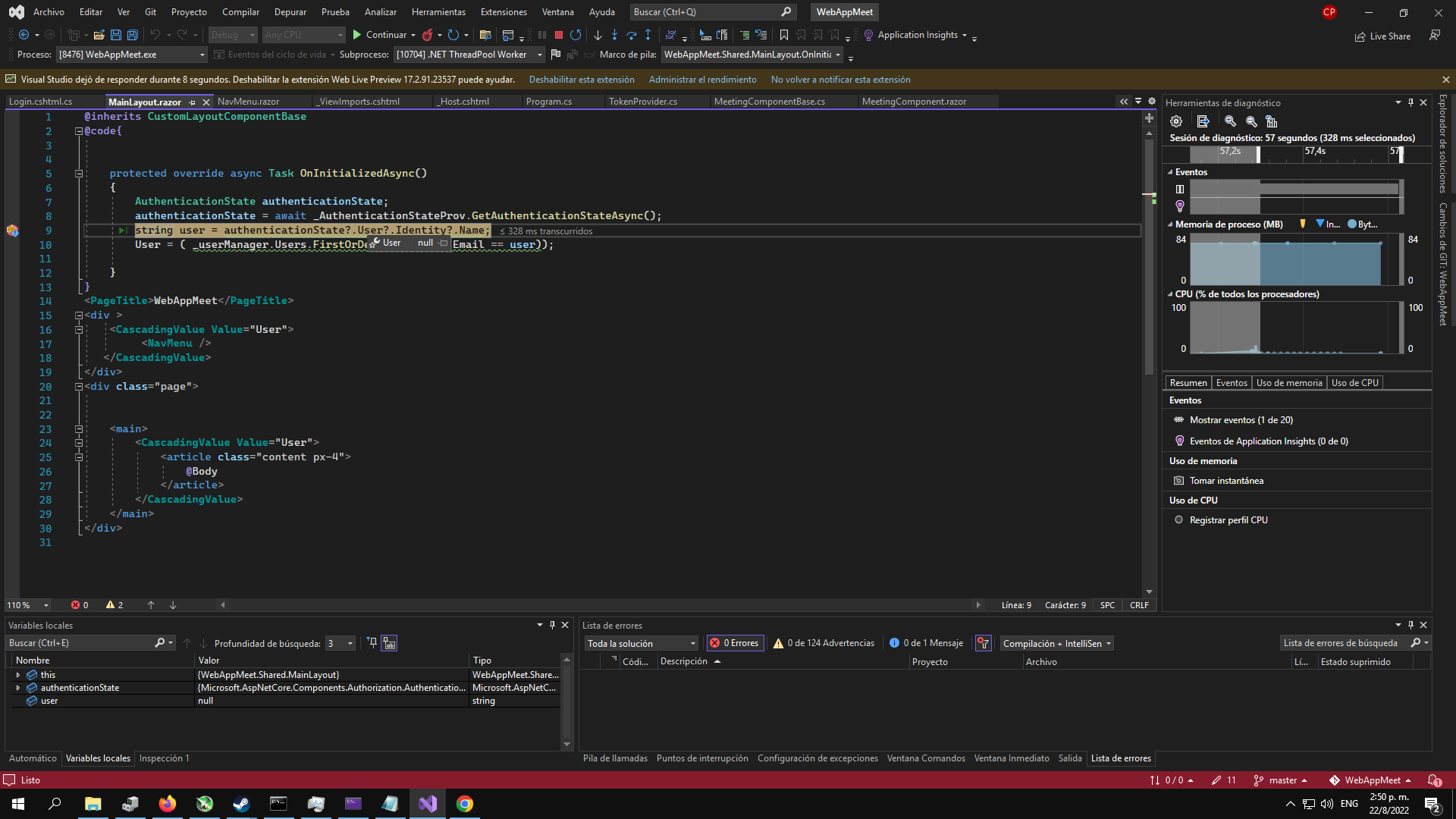
with identity scaffolding
builder.Services.AddAuthentication(options => {
options.DefaultAuthenticateScheme = "Identity.Application";
options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
})
.AddCookie()
.AddJwtBearer(options =>
{
options.RequireHttpsMetadata = false;
// Configure the Authority to the expected value for
// the authentication provider. This ensures the token
// is appropriately validated.
options.Authority = "/Security/Token/Validate"; // TODO: Update URL
// We have to hook the OnMessageReceived event in order to
// allow the JWT authentication handler to read the access
// token from the query string when a WebSocket or
// Server-Sent Events request comes in.
// Sending the access token in the query string is required due to
// a limitation in Browser APIs. We restrict it to only calls to the
// SignalR hub in this code.
// See https://docs.microsoft.com/aspnet/core/signalr/security#access-token-logging
// for more information about security considerations when using
// the query string to transmit the access token.
options.Events = new JwtBearerEvents
{
OnMessageReceived = context =>
{
var accessToken = context.Request.Query["access_token"];
// If the request is for our hub...
var path = context.HttpContext.Request.Path;
if (!string.IsNullOrEmpty(accessToken) &&
(path.StartsWithSegments("/ConnectionsHub")))
{
// Read the token out of the query string
context.Token = accessToken;
}
return Task.CompletedTask;
}
};
});
Security considerations in ASP.NET Core SignalR
Learn about security in ASP.NET Core SignalR.
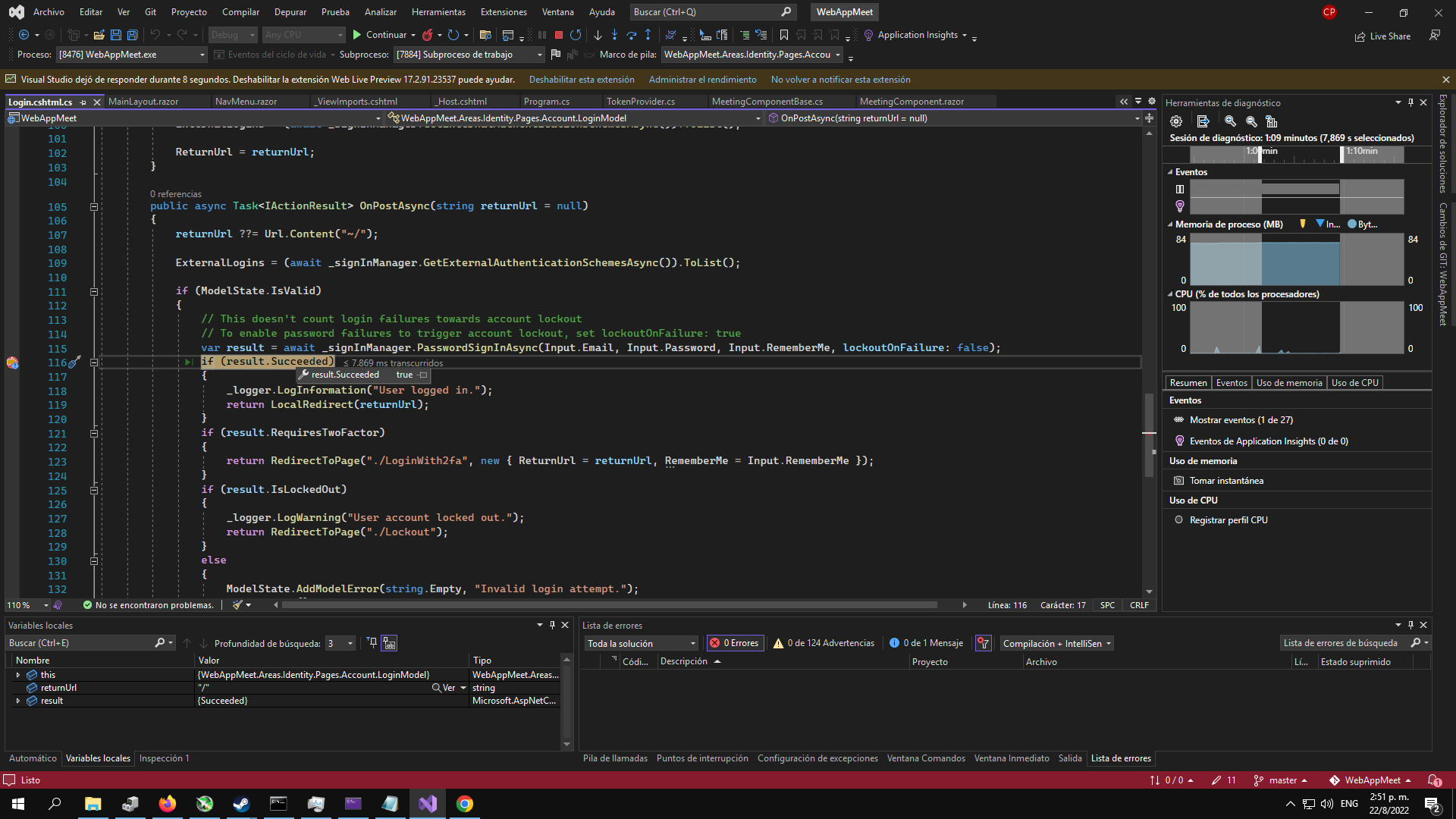
so after this the sesison is not givign me any identity
this happens if i did this
i do assume that
i need to pick either of these
options.DefaultAuthenticateScheme = "Identity.Application";
options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
found this https://weblog.west-wind.com/posts/2022/Mar/29/Combining-Bearer-Token-and-Cookie-Auth-in-ASPNET
Combining Bearer Token and Cookie Authentication in ASP.NET
In some situations you might need to use both Bearer Token and Cookie Authentication in a single application. In this post I look at a few scenarios where this is required and show how to configure your Authentication to let you access your site with either authentication scheme.
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
well
if i ask for a cookie on the identity authentication
i dont know how i would make the request for authenticating in the server side
to hold the identity in the client
so i think i need the cookie
and for the use of certain pages use the JWT
or if i enable JWT will identity hold the identity in the downloaded dlls?
i mean if i enable JWT apparently removes the identity values
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
but using only cookies i can access the AuthenticationState
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
I need to access
the User.Identity object because
it is how i can do some sort of authorization on the pages
so if I select JWT and i will need requesting an authentication for each time i need to call USER.identity?
Or can I simply call Identity without asking for a JWT request on my api ?
because blazor uses AuthenticationState
Patrick God
YouTube
.NET 6 Blazor 🔥 Authentication & Role-Based Authorization (using JW...
🔥 Blazor E-Commerce Course: https://www.udemy.com/course/blazor-ecommerce/?couponCode=YOUTUBE
📧 Newsletter: https://mailchi.mp/364b891b448f/dotnetdev
❤️ Ko-fi: https://ko-fi.com/patrickgod
☕ Coffee: https://www.buymeacoffee.com/patrickgod
💻 GitHub: https://github.com/patrickgod/BlazorAuthenticationTutorial
🐦 Twitter: https://twitter.com/_Patrick...
apaprnetly if i use JWT i need to do a custom AuthorizationState classs
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
@PontiacGTX The idea behind Jwt is that everytime you make a request, you pass in the token which contains information about the user. You then use that data to authorize or access the user.
For the issuer and audience. Depending on if you have configured them both to be included than you would need to pass in some values when generating a token. Now for what you pass in, is up to you.
Now I saw here and there some things about signalR. When it comes to WebSocket. Authorization doesn't work the same way. Because there is no headers this means you cannot pass in the token when connecting to the socket or making your call. You will need to pass in the token as a parameter. Also I don't really understand what your main problem is right now?
getting the Auth to work with JWT
but apparently i need to do the implementation from the video