BinaryReader Question
Trying to read the values AS bytes, but reader reads them as decimals, is there a trick to making it bytes?
18 Replies
Just read the bytes directly from Stream
You don't need BinaryReader, that's for reading bytes as other types
hmm
but the issue i have is it comes back as decimals
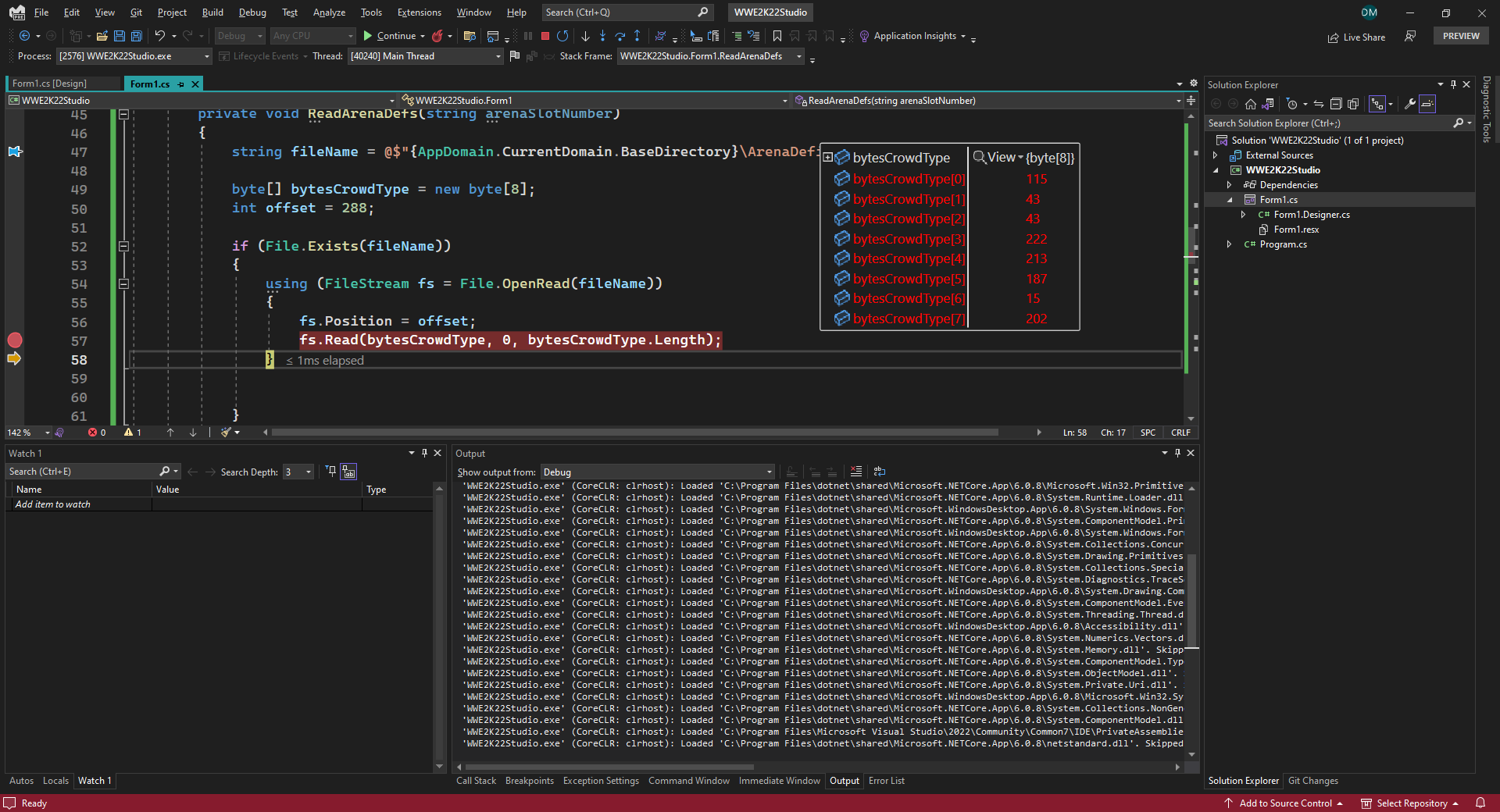
is there a way to convert it to bytes in the bytes[] array or it has to be .ToString("X") for that?
It is bytes
It's just displayed as base 10
Everything is bytes internally
That's just how the debugger is configured to display them
ah
Is there a way to turn them into this?
732B2BDED5BB0FCA
which is those in hex
Probably, google "visual studio debugger hex"
ty
wait, so if i put it to "RELEASE" it will show hex?
in my form
Why would release have anything to do with anything?
DebugOutputContent.Text = fs.Read(bytesCrowdType, 0, bytesCrowdType.Length).ToString("X");
Because I want the USER to see hex
no one makes games or mods or does stuff in base 10 🙂
even in Unity
I just suck at this I guess 😦
Release doesn't have anything to do with how you format things to display to the user
But yes if you want to display a hex string to the user you have to convert the bytes to a hex string like that
foreach (byte b in bytesCrowdType)
{
DebugOutputContent.Text += b.ToString("X");
}
got it 🙂
Thank you for putting up with my dumb question
I appreciate your time and help
Also the new help post system is amazing 🙂
Yeah I kinda like it lol
It's nice
Yeah Discord did g ood on that front 🙂
Last question based on 'writing' bytes (fs i guess)
don't worry if its not hex then right?
it will convert to hex in file?
If you want to write bytes you write bytes from a byte array
Make sure you don't write a string
so like this
someone told me you needed to loop through each byte in the array too
Why are you seeking and writing one byte at a time?
Just seek once and write the whole array?
I am not sure how to do that with writing
fs.Position = offset;
fs.Write(bytesCrowdType);
ah
think i got it