Signalr not sending message(s) to specific user(s)
in the hub i have setup this signature for a method
and in the client side(the page on blazor server) i have set this up for the ReceiveMessage method
and how I call this method
68 Replies
@PontiacGTX Right now, what is your problem? Your message isn't received by the user, or it's received by too many users?
it isnt recieved by me (who sent it)
it moves into the SendAsync method in SendMessage
and then it doesnt trigger the Action
(I am the sender and the receiving user)
Have you debugged the service for when you connect with the client to make sure that part works, and when you try to send a message to the user, what's the value you're using for it's client?
some ranodm string
but i see that the client is repeated twice
maybe i shoudl use an if else
A random string? How do you expect that to work?
if it is the use myself only send it to me and not twice my username
i mean the message is what i have sent
(some random thing) lol
Ok, but I'm talking about the recipient. What's the value?
You're actually sending the message twice, once to the recipient and once to the sender.
my username
in identity
i am senidn ti twice yeah
but not receiving it once
Then that's probably the problem. SignalR doesn't care about your identity itself, it wants the clientId.
mmm
then this doesnt work ?https://stackoverflow.com/a/63771575/13794072
Stack Overflow
SignalR - Sending a message to a specific user using (IUserIdProvid...
In the latest version of Asp.Net SignalR, was added a new way of sending a message to a specific user, using the interface "IUserIdProvider".
public interface IUserIdProvider
{
string GetUserId(
await _userManager.AddClaimAsync(user, new Claim(ClaimTypes.Email, Model.Email));
this line
Did you create and register the
CustomEmailProvider
?
(And is the email part of the claims of the user?)well i dont know if i setup the custom email provider
where do i set it?
It's in the SO post.
i have this
then
builder.Services.AddSingleton<IUserIdProvider, CustomEmailProvider>();
builder.Services.AddSignalR();
Oh, then you have the custom email provider. If you put a breakpoint in there, what happens?
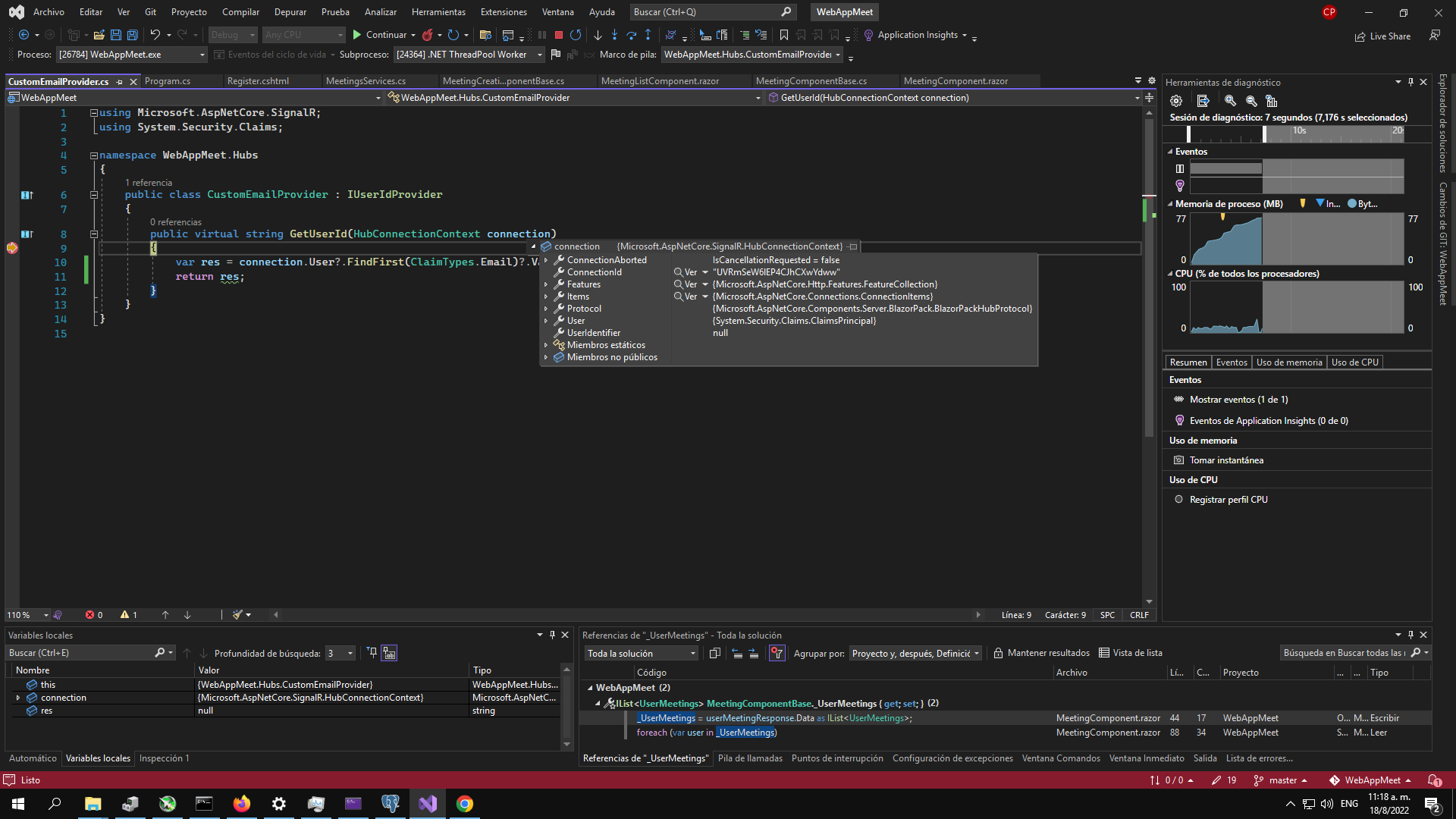
just starting up
(i havent sent anything)
Humn... what's the value of res?
loggged int
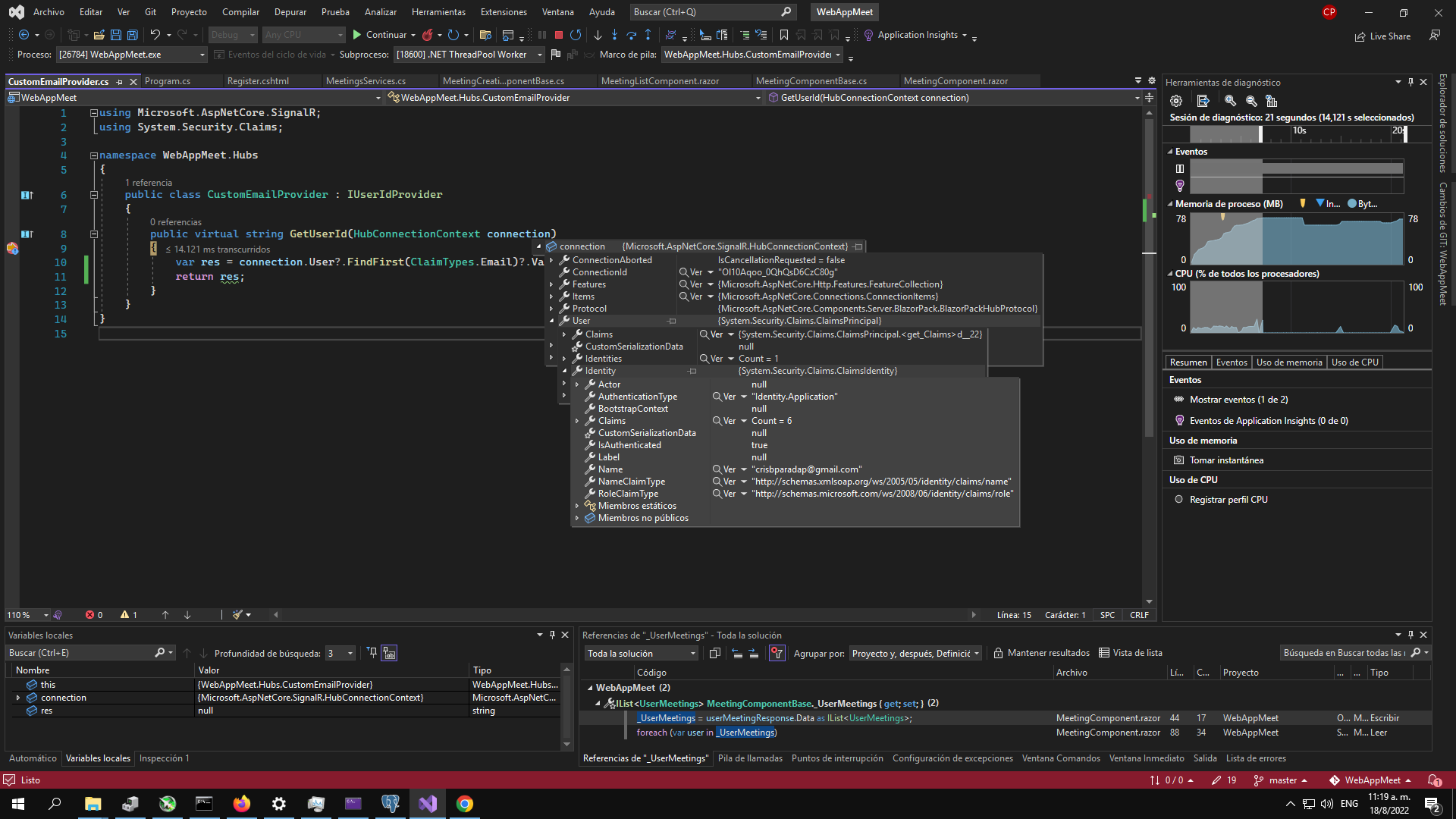
it skips it
i think this is wrong
let me try something different
var res = connection.User?.Claims.FirstOrDefault(x=>x.Type==ClaimTypes.Email)?.Value;
this worked somehow the other method didnt lol
Good. Then
res
is a Guid (well, string representation) and your message gets sent?it si my username
so my email
That's not what you want.
why??
oh well
i see the method says
the Id
connection User
contains the id
also i would like to use my email a smy idnetifier
but if you say i must return the id...
Well, I might be wrong. I always used the plain SignalR UserId. Give me a sec to read on it.
OH! Nevermind, it's now how I thought it was.
It must return the email, you're right
What it's doing is not "map the email to the userId". It's, when a connection is established, SignalR asks that provider "What do you want me to use as Id"
So to recap: This must return your email in your context.
well in my context let me check
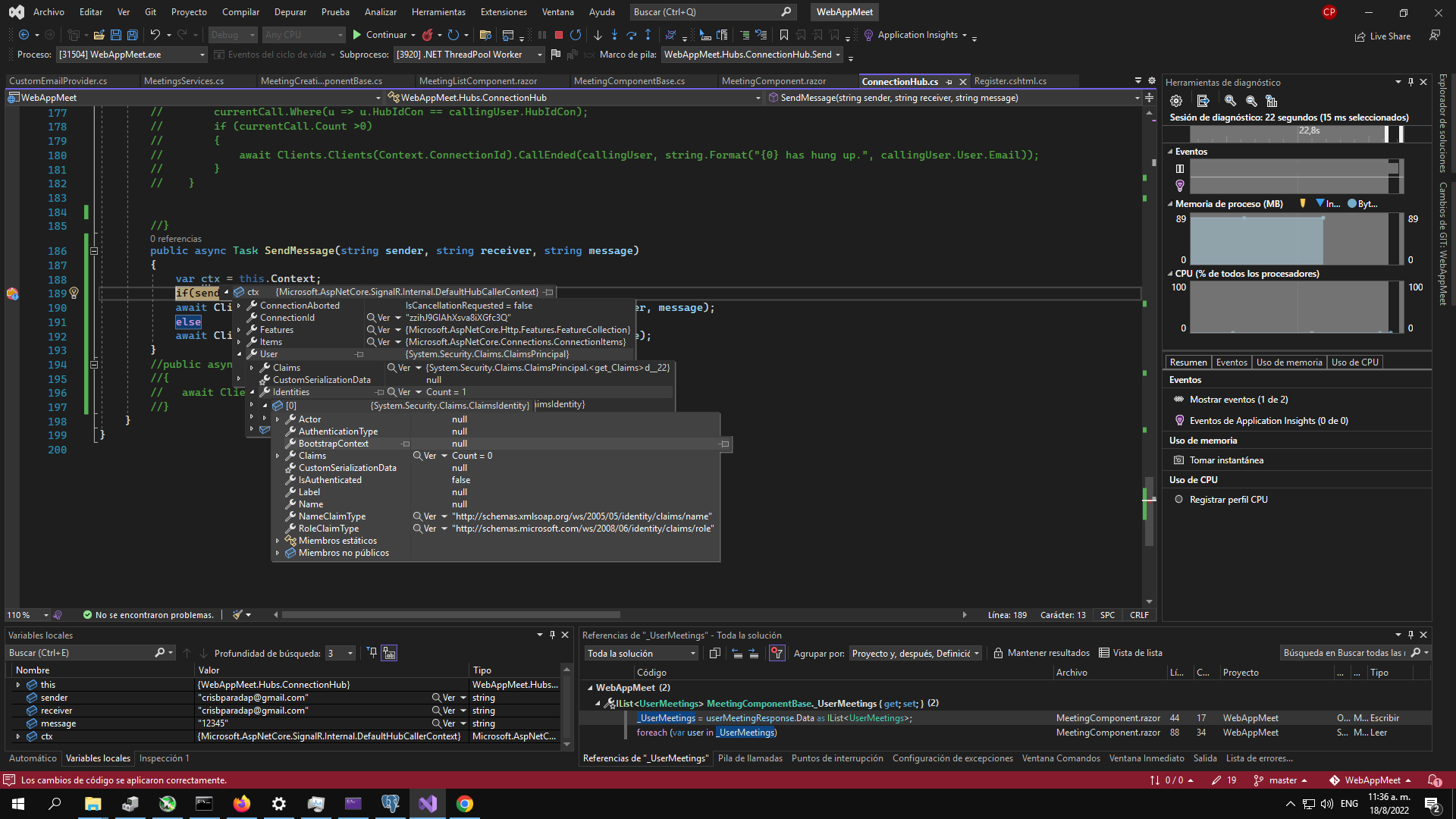
this si what the context returns
no user
But you're not in the same context there.
where do you want me to check the context
In the code of the UserIdProvider, it's when you're establishing the connection as the user.
There, you were sending the message.
In
GetUserId
, the value returned is the email now, right?
(if you put a breakpoint and check when you establish the connection.)i mean it does only when I login
Of course
i have a cascade parameter on my nav
and on my body fragment
but wheni click to send the message it just doesnt moves onto GetUserId
Wait, you're losing me. What are you talking about now?
No, it's ok. It's when the SignalR connection is established only.
I am talking about triggering the SendMessage method
oh well
so where do i check that the user is within the context
it isnt in the hub on the server?
.
Yawnder#7904
In
GetUserId
, the value returned is the email now, right?Quoted by
<@!163876962710847488> from #Signalr not sending message(s) to specific user(s) (click here)
React with ❌ to remove this embed.
it does
Great
but then it doesnt really send the mssage
Not at that moment no. Now, when you send the message, you send the same email address as recipient, correct?
yeah who is myself
who sends it
And you still don't receive messages, right?
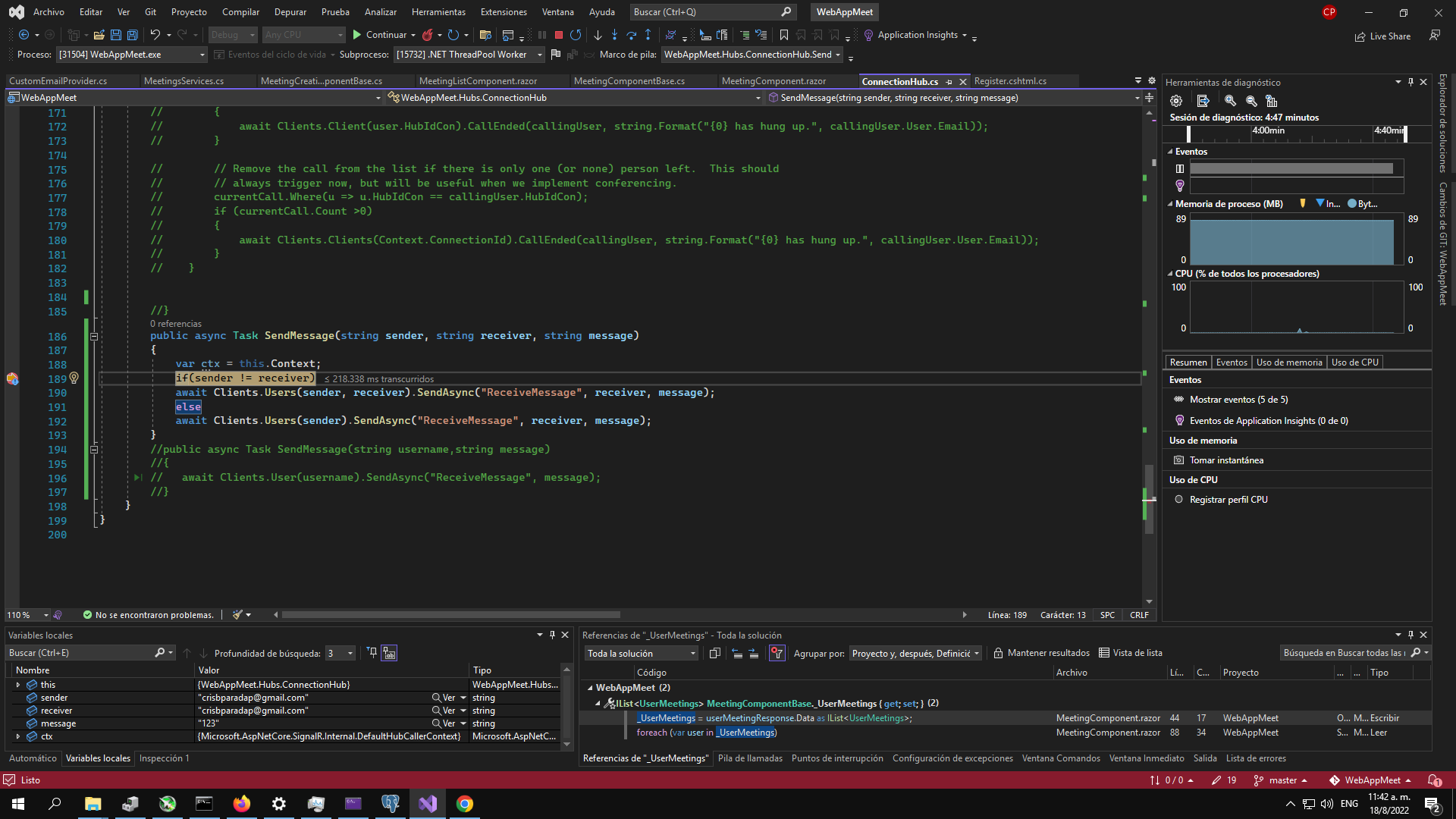
i dont recieve anything
i wrote it like this
and nothing triggers this
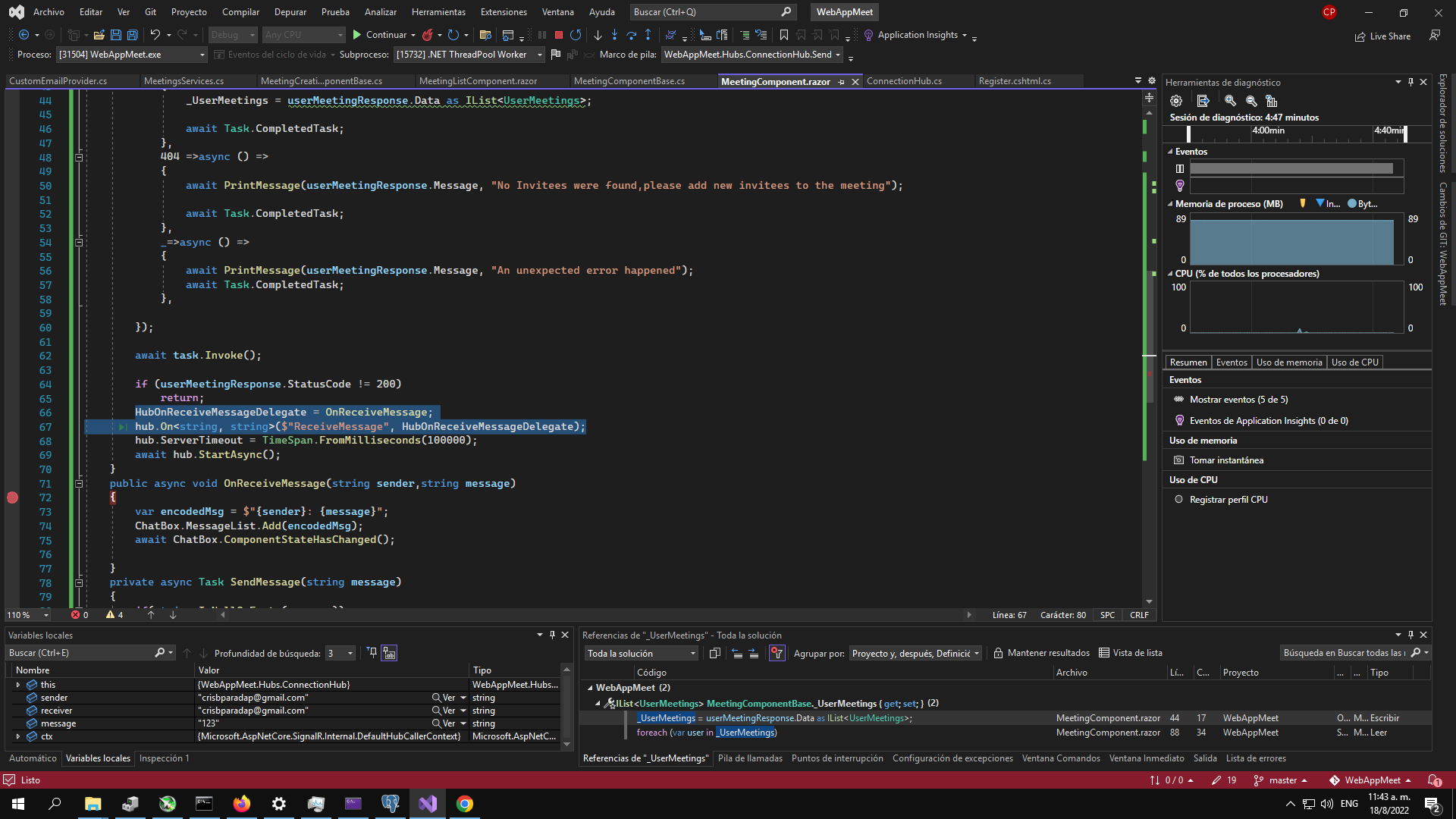
this action doesnt trigger this method
You have a breakpoint in
HubOnReceiveMessage...
and it doesn't get hit? Okit doesnt
In your hub, add this override and put a breakpoint in there:
public override async Task OnDisconnectedAsync(Exception exception)
Also, in SendMessage
, can you check the content of Clients
and see if you still have some.ok
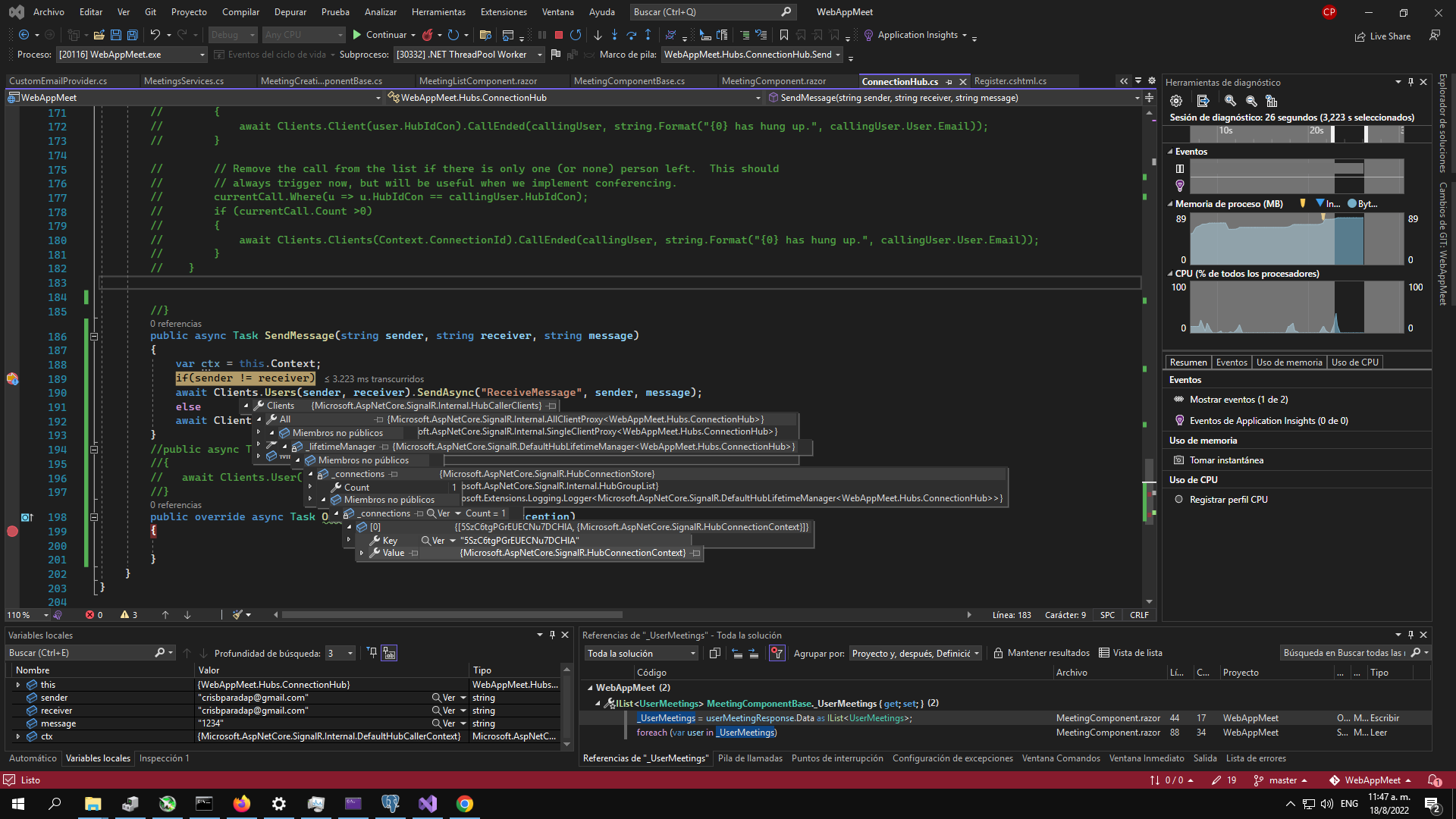
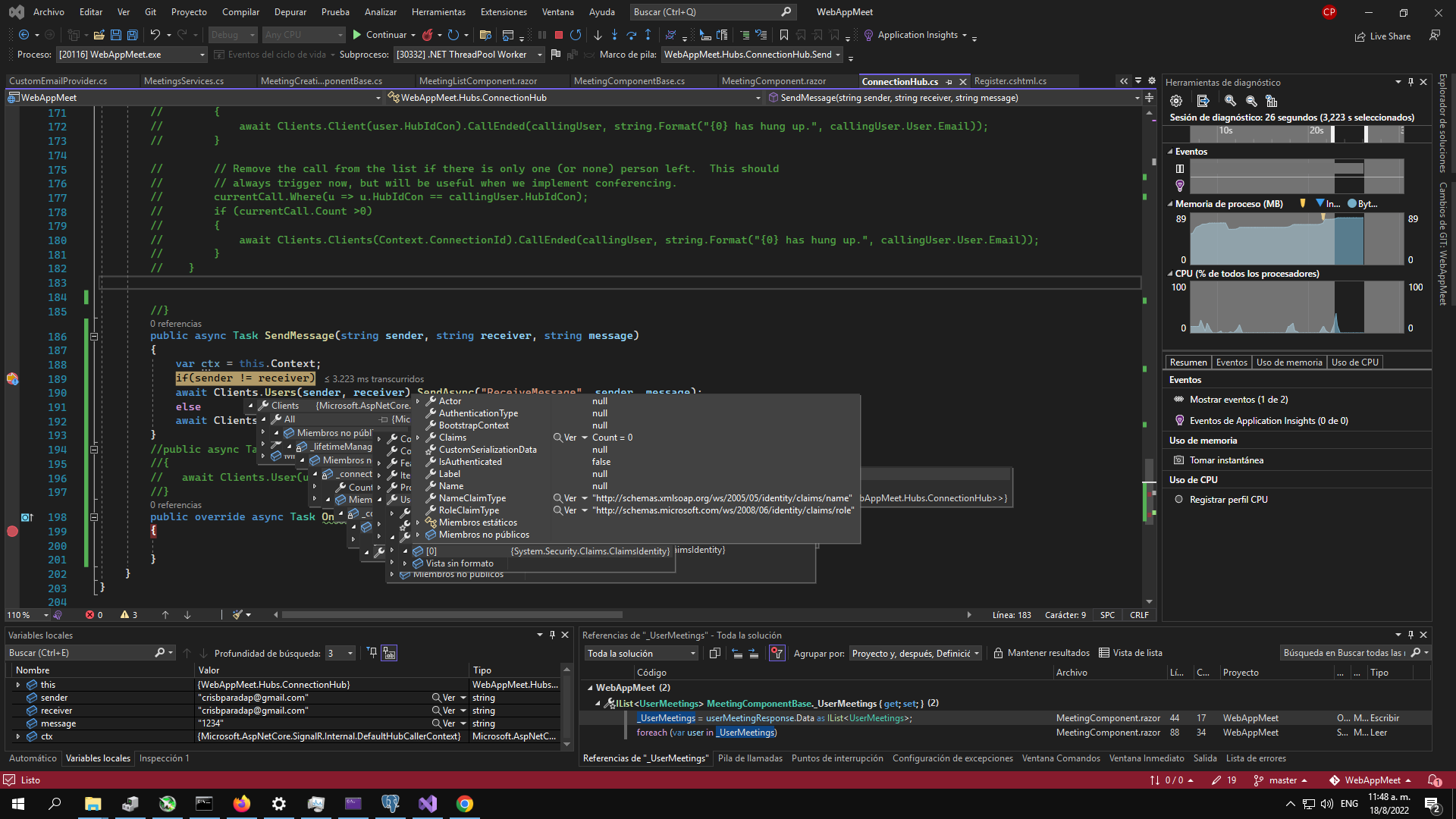
user seems null
If you send to
Clients.All
, does it work?i will restart again and check that out
yes it works
so why it isnt binding the user to the email
Then I don't know. I would have to try it and play around
can i send you my repository?
I don't really have the time for that, sorry
so what can I do to make sure that it beinds the userid to the email
No idea. As I said, I always worked with the raw UserId. Maybe if you put a breakpoint in SendMessage and you check
this.Users
to see what's in there.this.Clients?
There is no list of users?
Users isa method
that calls certain users
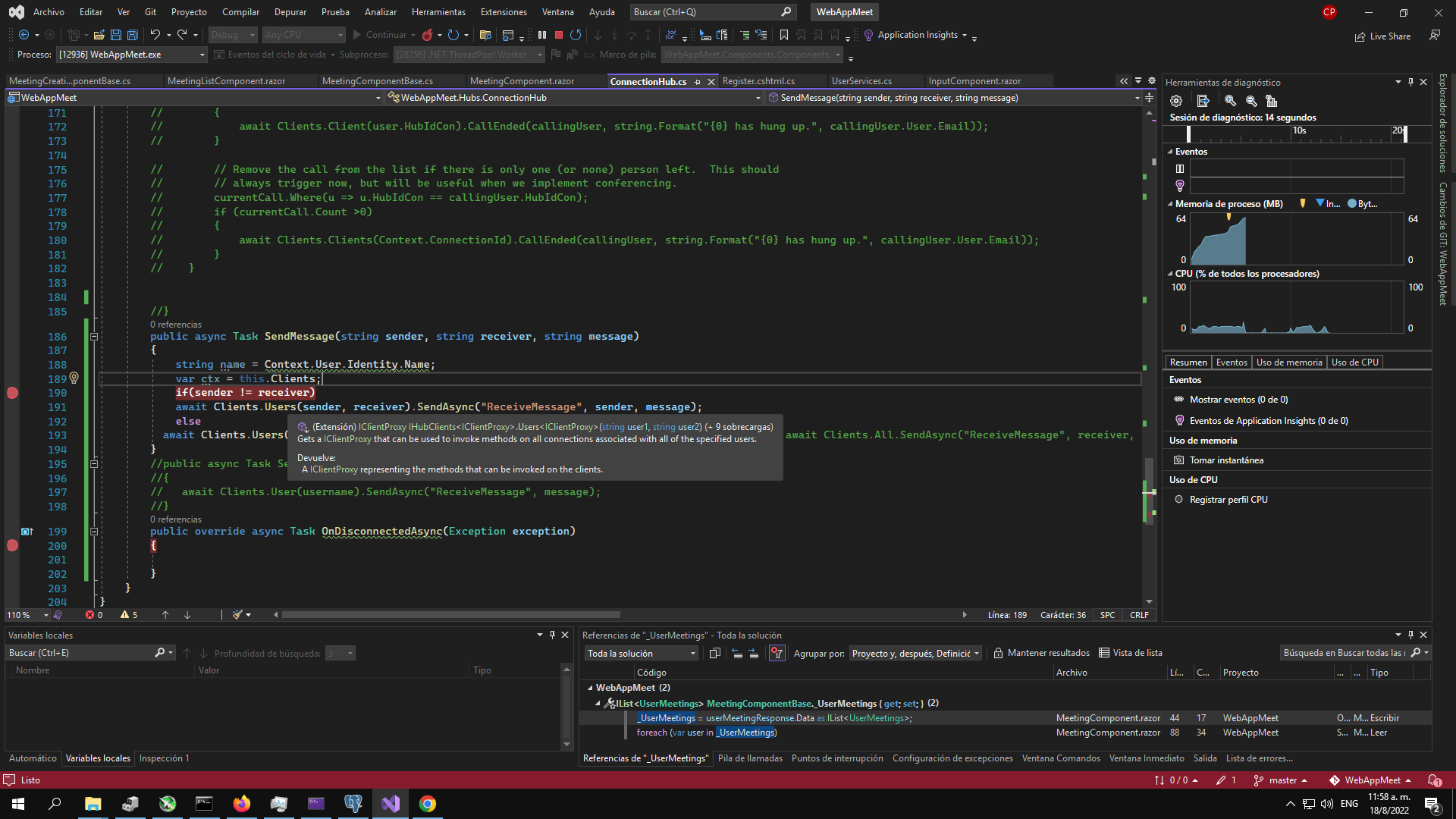
there is a connection
but no claims or users