Wpf Button CommandParameter
<Button Command="{Binding ClickCommandEvent}" CommandParameter="Jack"/>
How commandParemeter value get wpf ?
20 Replies
A command parameter is the same as other dependency properties. You bind it to the value you want to pass, otherwise it will pass as a string
And then assuming that your ClickCommandEvent is an ICommand you can receive that parameter through the Execute methods parameter
@frknztrk you should use
CommunityToolkit.Mvvm
, it provides all the basic stuff you'll ever need.i want a simple build
It can't get any simpler than using
CommunityToolkit.Mvvm
. It provides a ready-made RelayCommand
/ AsyncRelayCommand
implementations, as well as generic versions RelayCommand<T>
/AsyncRelayCommand<T>
(which are the ones you want when using CommandParameter
)
Docs reference: https://docs.microsoft.com/en-us/windows/communitytoolkit/mvvm/introduction
Feel free to write everything on your own, but this would both save your time and provide best-in-class implementations.What I want is to get the CommandParameter data when I click the button in the dynamically created data source.
Mainwindow.xaml
MessageViewModel.cs
Person.cs
MessageCommand.cs
Seems like what you wrote here should work, though I think your message command class is gonna have problems
Yeah, your
MessageCommand
is basically ignoring the string
parameter.Huh?
I was referring to him not connecting back to CommandManager
ICommand and RelayCommand in WPF
This article provides a basic overview of commands in WPF. Here ICommand RelayCommand will be discussed.
Only because I can't figure out where more official docs are
I believe your mistaken
Oh well. Can execute isn't checking the param
Works when using outside of data template
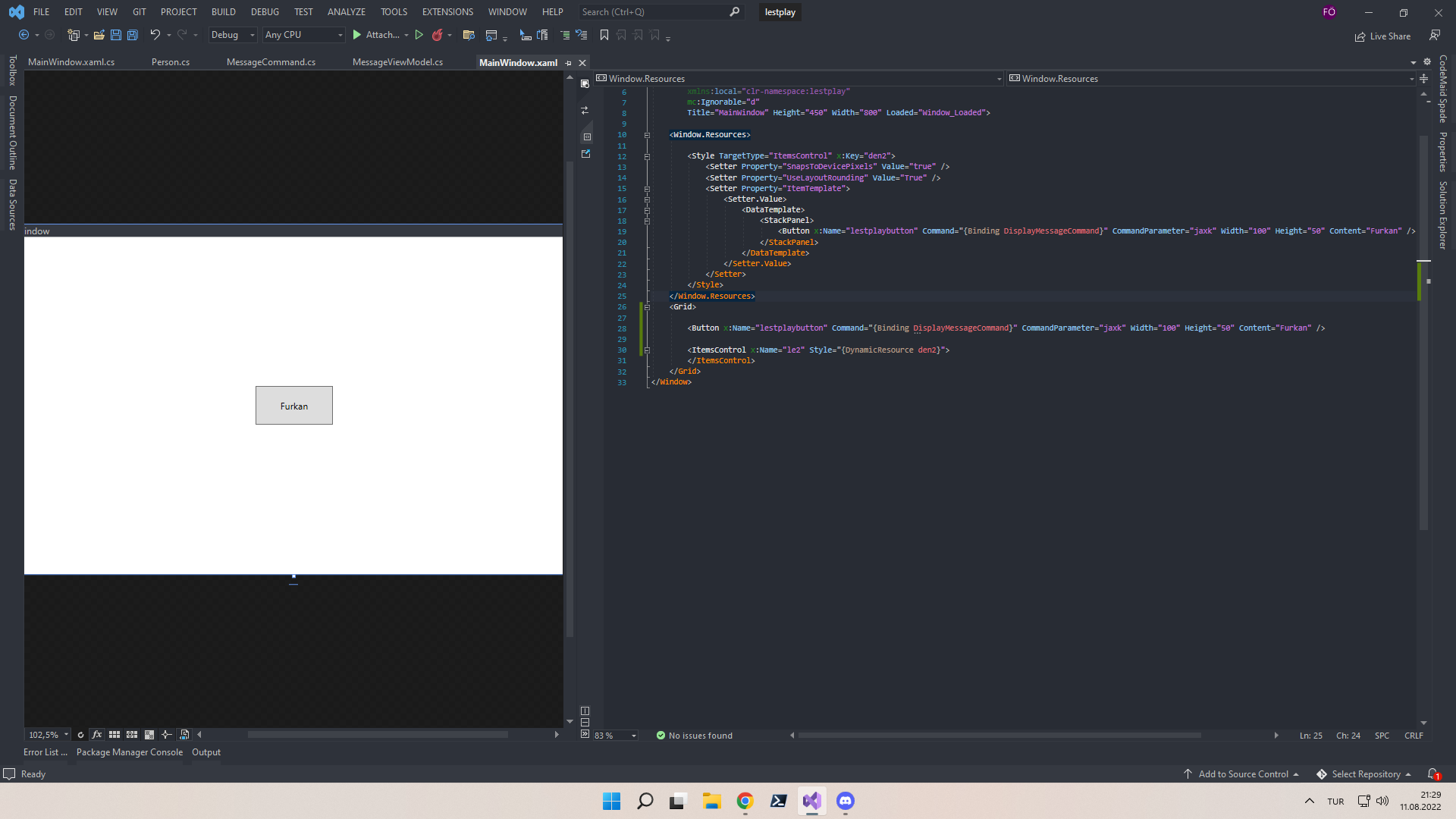
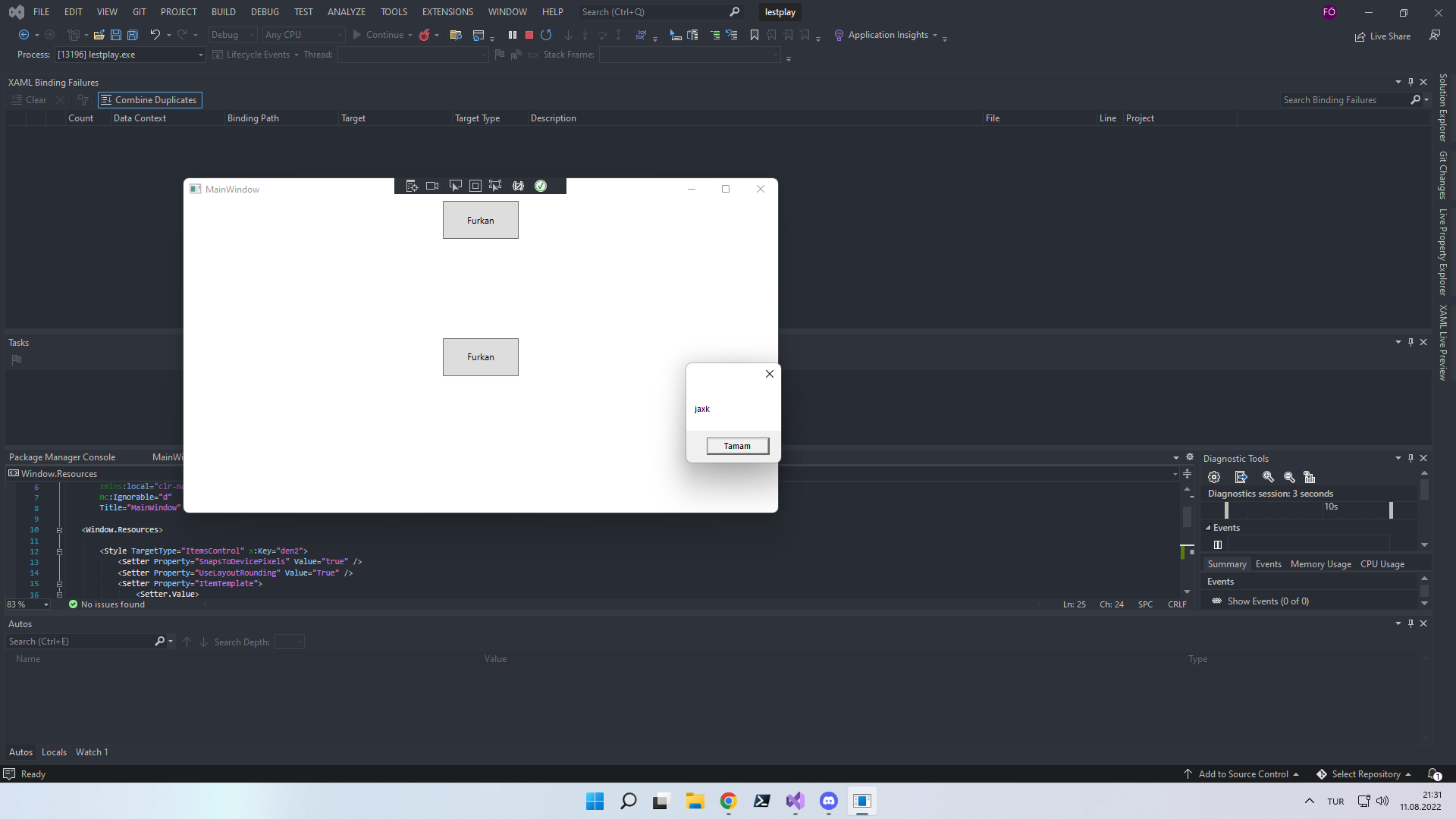
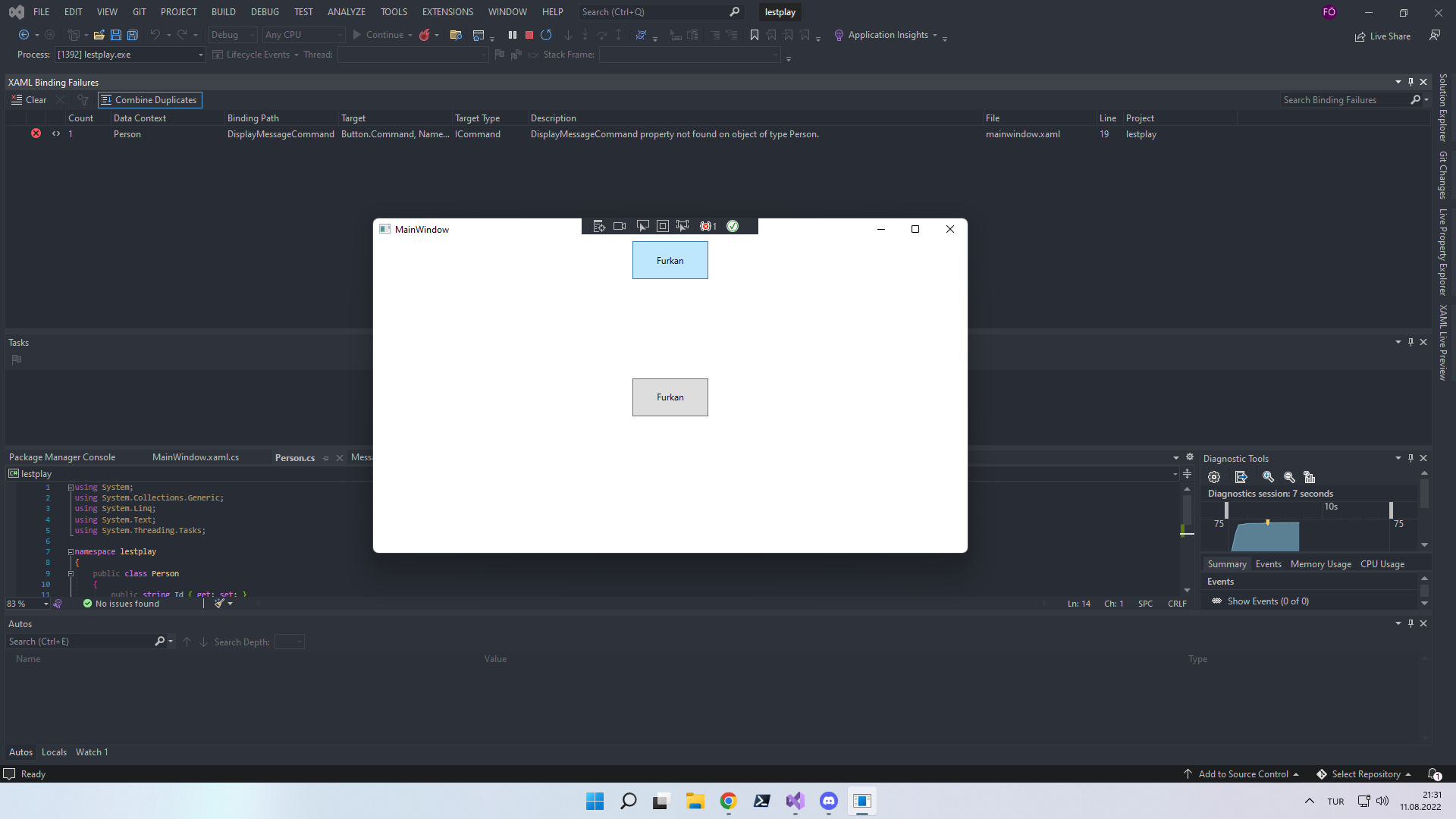
The issue is that your
Command
binding is incorrect. It doesn't have to do with the CommandParameter
.
I suggest not putting the <DataTemplate>
into your resources if you can avoid it, so you can have a simpler ElementName
binding instead of RelativeSource
ancestor binding.
Currently, the binding is looking for the command on Person
because that's the local DataContext
within the <DataTemplate>
.
This is how I would rewrite it so the Button
command works. I'd probably get rid of the style altogether.I think it's okay, thank you, but I have to use style because I'm getting my data dynamically.
but why does it not see the element name in such a render
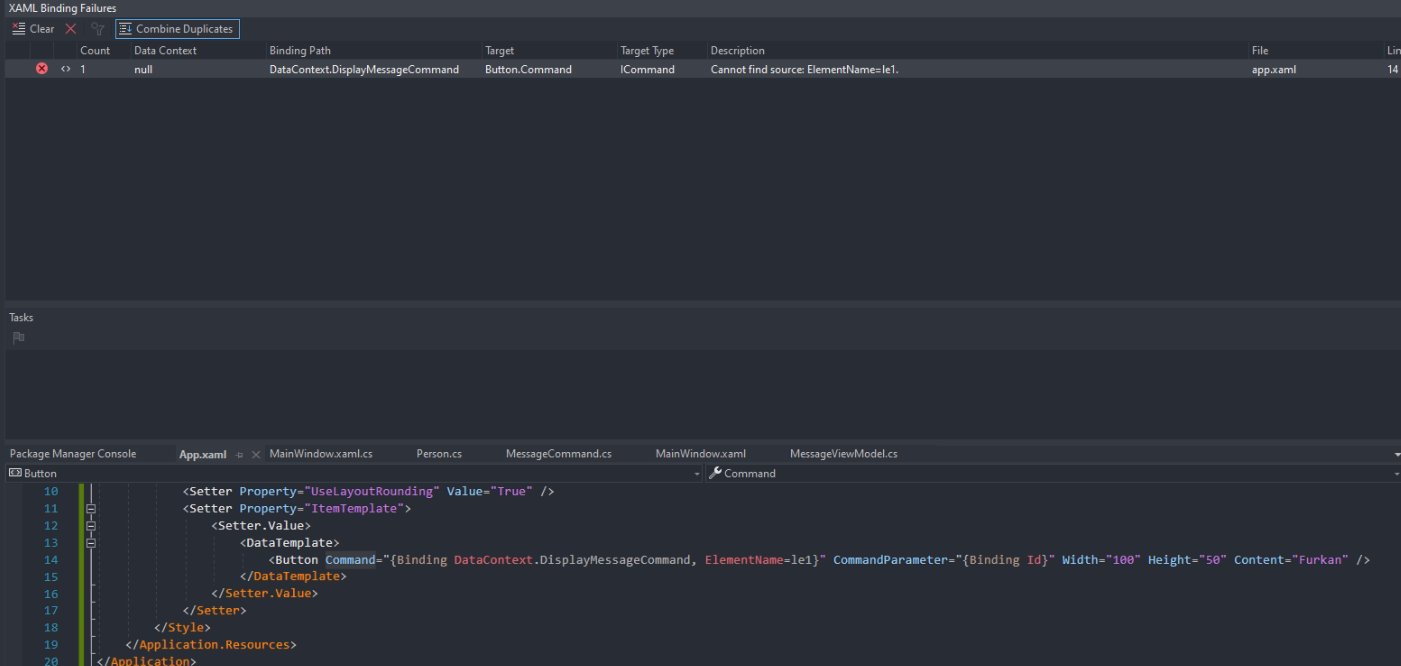
Because you didn't follow the advice. There's no reason this needs to be a style. You can't access element names like that when you're in
.Resources
, AFAIK.
Which means you now have to write a RelativeSource
ancestor binding which looks something like:
sorry i am new to .net i am missing some points
It's a complicated binding, but you need that indirection if the data isn't located on the current
DataContext
.