sapphire-support
discordjs-support
old-sapphire-support
old-discordjs-support
old-application-commands-and-interactions
Check if command is a slash command
command.supportsChatInputcommands()
https://www.sapphirejs.dev/docs/Documentation/api-framework/classes/Command-1#supportschatinputcommands...Is it possible to send voice messages from a bot?
Error [EMPTY_MODULE]: A compatible class export was not found.
interaction-handlers
directory (create it if you don't have one) and the issue should be solvedPaginatedMessages
/tag query: bots
from @Spinel to get a long list of open source bots. Some use PaginatedMessage. Plenty of examples to go off of....Automod Issue
Dyno like help command
!<commandName> help
it displays the help embed instead of having to run !help <commandName>
help
subcommand which displays the detailed descrition. You would then have all your other commands extend that class to inherit the help
subcommand.i18n based on the user locale
resolveKey
or something else??command failing, no errors
Slash command not working
ReferenceError: exports is not defined in ES module scope
@sapphire/*
because otherwise TS cannot properly resolve module augmentations. I forgot the exact option but it's one of the hoist pattern options.
As for your root cause, it really comes down to how you configured tsconfig. The modern standard is to use node16
for both module
and moduleResolution
then either use "type": "commonjs"
or "type": "module"
in your package.json based on whether you respectively want a CJS or ESM runtime.
Once that is done and you compile with either exclusively swc (which you seem to use yet you also mention ts-node, they DO NOT work together, also we discussed the downsides of ts-node) or alternatives such as tsc
/ tsc-watch
/ tsup
....props to button
Multiple listeners on the same event ?
chatInputCommandError
)
My folder is organised as such:
src >
listeners >...{ event: '...' }
into the constructor (either via @ApplyOptions
from @sapphire/decorators
or with super()
)ChatInputCommandInteraction
ChatInputCommandInteraction
, I tried importing it from the framework, however is returned this: TypeError: Right-hand side of 'instanceof' is not an object
I'm trying to do this:
I have a createError
function, which sends a embed with the provided text and then deletes the message and the embed after some seconds, however I want it only reply if the variable is a interaction instance.
...Issue with code not running after using args.rest('string') (NOT SAPPHIRE ISSUE)
how to make fetchPrefix return a regex?
messageSubcommandNoMatch listener not working
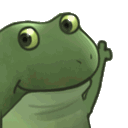
messageSubcommandNoMatch
error. I've registered a listener for it:
listeners/messageSubcommandNoMatch.ts
```ts...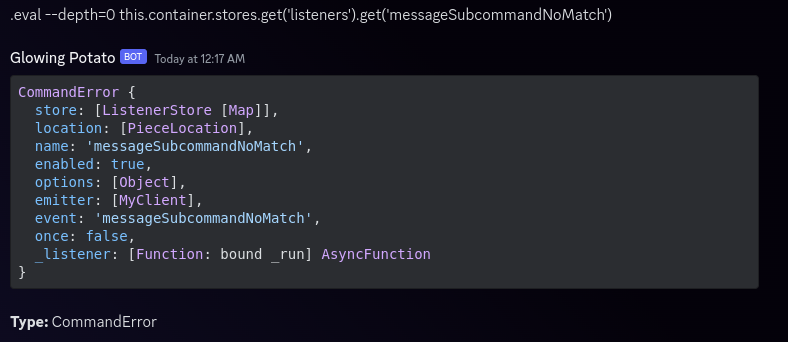
Invoking Command method from other command
this.store
, just make sure you pas all the right arguments:
```ts
import { Args, Command, type MessageCommand } from '@sapphire/framework';
import type { Message } from 'discord.js';...error
TypeError: Cannot read properties of undefined (reading 'parseConstructorPreConditionsRunIn')
TypeError: Cannot read properties of undefined (reading 'parseConstructorPreConditionsRunIn')
Register a command on every guild
registerApplicationCommands
in the command that is. Then as for how it will help, you can register the commands yourself to the guild in question when you receive a guildAdd event.Intent question- paginatedmessage